Children props
FabrixComponent
provides useful functions and data through its children prop. Once children prop is given to FabrixComponent
, the React node returned from children completely overrides how the component is rendered.
This feature is expected to be used to decorate the component or modify styles of it in an ad-hoc way. For more precise control like in-component styling, callback and behaviour, you can use the custom component builder.
Anatomy
The terminalogies used in functions that children prop provides are coming from as follows:
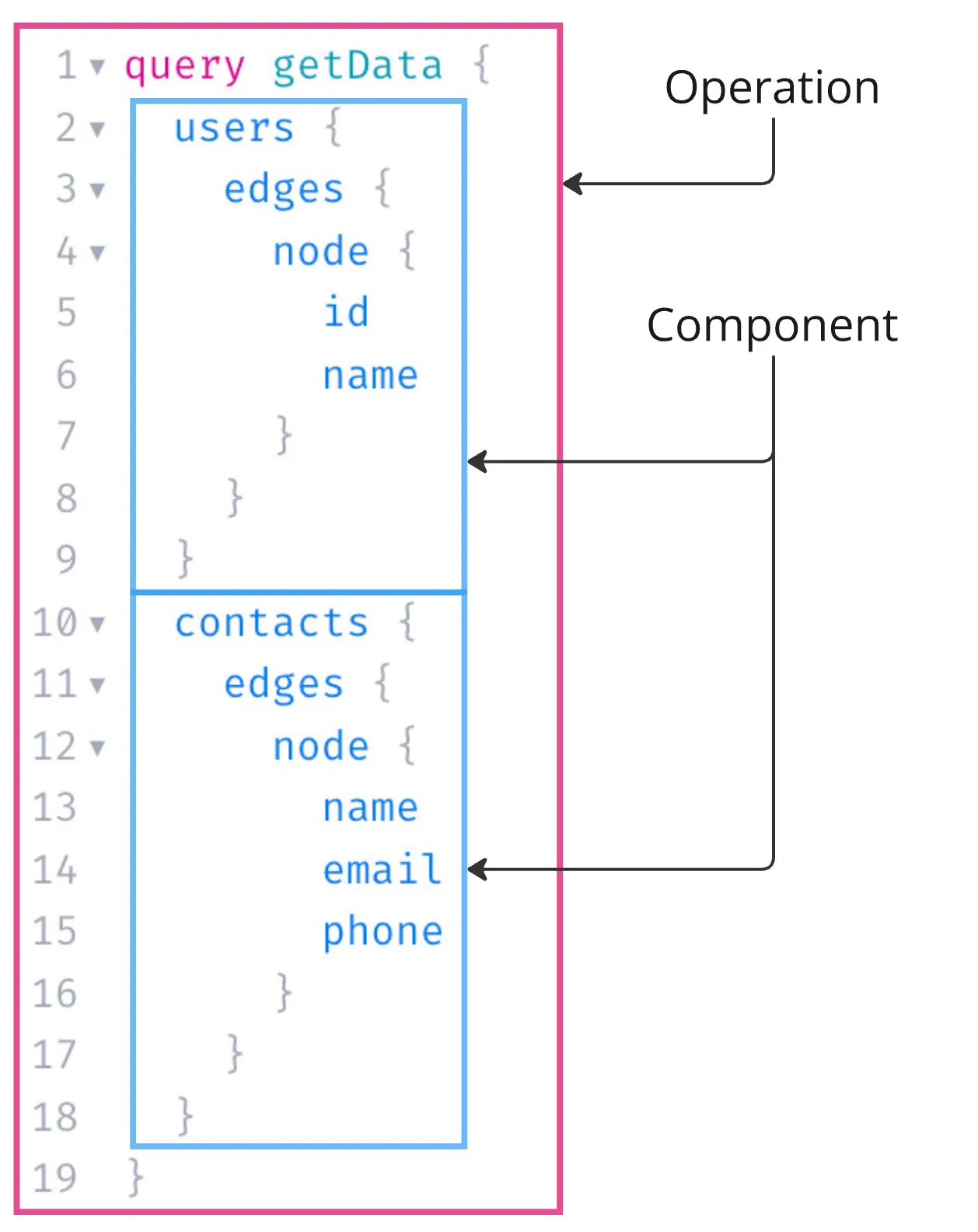
getComponent
getComponent
is a function that renders a component associated to the operation and the included selection.
Fabrix renders components based on the selection in a GraphQL operation, so this function is the minimum unit to render a component.
<FabrixComponent query={` query getData { users { edges { node { id name } } }
contacts { edges { node { name email phone } } } } `}> {({ getComponent }) => ( <> <h1>Company list</h1> {getComponent("getData", "contacts")} </> )}</FabrixComponent>
getOperation
getOperation
is a function that renders a component associated to the operation name.
Fabrix supports multiple operations in query
prop, so with this function users would be able to render components selectively.
<FabrixComponent query={` query getUsers { users { edges { node { id name } } } }
query getContacts { companies { edges { node { name email phone } } } } `}> {({ getOperation }) => ( <> <h1>User list</h1> {getOperation("getContacts")} </> )}</FabrixComponent>
Plus, getOperation
has a render prop in the second argument that gives us getComponent
as well.
<FabrixComponent query={/* same query as the example above */}> {({ getOperation }) => ( <> <h1>User list</h1> {getOperation("getUsers", ({ getComponent }) => ( getComponent("users") ))} </> )}</FabrixComponent>
data
data
is a raw value that is simply passed down from the fetched result.
<FabrixComponent query={` query getUsers { users { edges { node { id name email } } } } `}> {({ data }) => { /* * `data` has data as follows: * * data.getUsers.users.edges[0].node.{id,name,email} * data.getUsers.uesrs.edges[1].node.{id,name,email} */ }}</FabrixComponent>